
Introduction to Python Programming
Python is a versatile and powerful programming language that has recently gained immense popularity. Whether you are a beginner or an experienced programmer, learning Python can be a game-changer in expanding your coding skills and career prospects.
What is Python?
Python is an open-source, high-level programming language that focuses on code readability and simplicity. It was developed by Guido van Rossum and first released in 1991. Python emphasizes clean and easy-to-understand syntax, making it an excellent choice for beginners. It has a vast standard library and a thriving community that offers numerous resources and support.
Why should you learn Python?
There are several compelling reasons why learning Python is beneficial:
- Easy to Learn: Python’s simple syntax and readability make it easier for beginners to grasp the fundamentals of programming.
- Versatile: Python can be used for a wide range of applications, including web development, data analysis, machine learning, scientific computing, and more.
- In-Demand Skills: Python is one of the most sought-after programming languages in the job market. Learning Python will expand your career opportunities and increase your chances of landing lucrative job offers.
- Large Community: Python has a vast community of developers who contribute to its growth and development. This means abundant resources, tutorials, and support are available to assist you in your learning journey.
If you want to become a web developer, data scientist, or improve your problem-solving skills, learning Python will be beneficial in the future.
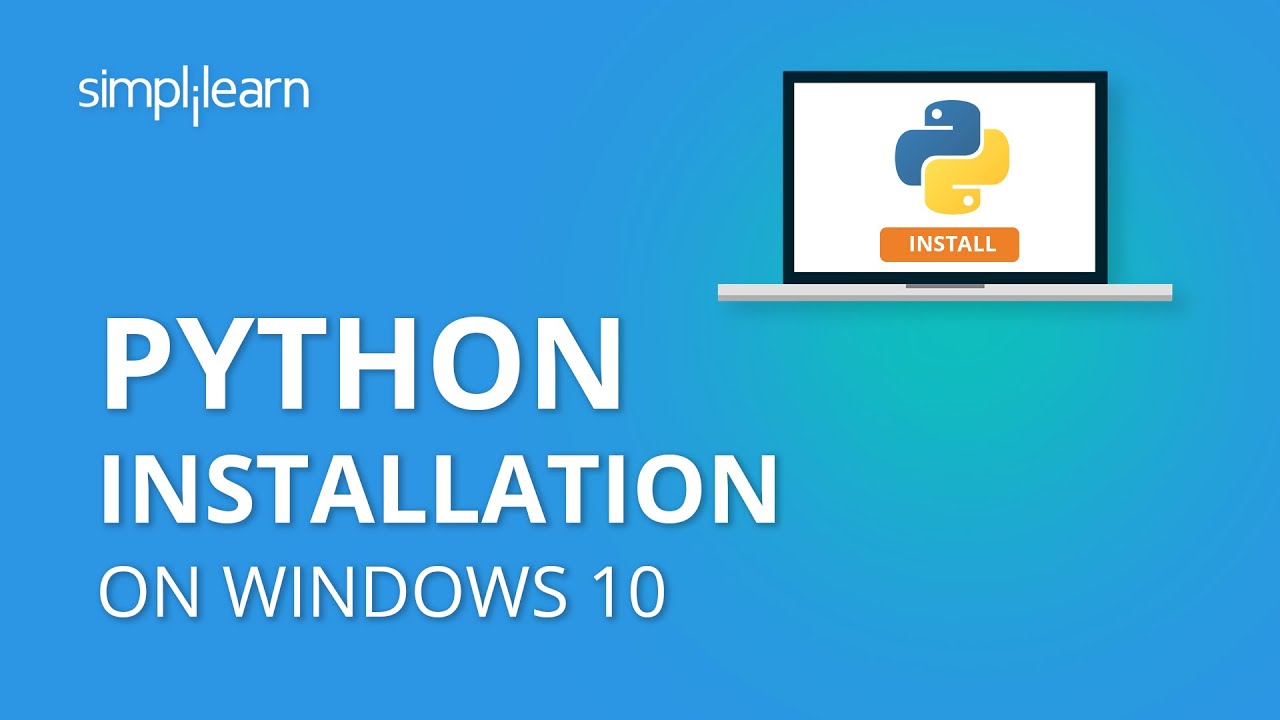
Installing Python and Setting Up the Development Environment
Python is a versatile and powerful programming language that is widely used in various domains, including web development, data analysis, and machine learning. If you are a beginner looking to learn Python, it is essential to start by installing Python and setting up your development environment.
Installing Python on different operating systems
Python is compatible with multiple operating systems, including Windows, macOS, and Linux. Installing Python on your preferred operating system is relatively straightforward:
- For Windows: Visit the official Python website and download the Python installer for Windows. Run the installer and follow the prompts to install Python on your system.
- For macOS: macOS comes pre-installed with Python 2.7. However, it is recommended to install the latest version of Python using a package manager such as Homebrew or Anaconda.
- For Linux: Most Linux distributions come with Python pre-installed. However, it might be an older version. You can update it using your package manager or install the latest version from the official Python website.
Choosing an Integrated Development Environment (IDE)
An Integrated Development Environment (IDE) makes coding in Python more convenient and efficient by providing features like code editors, debuggers, and project management tools. Some popular choices for beginners include:
- PyCharm: A feature-rich IDE developed by JetBrains, PyCharm offers a community edition that is free to use.
- Visual Studio Code: A lightweight and highly customizable code editor that supports various programming languages, including Python.
- Jupyter Notebook: Jupyter Notebook provides an interactive environment for writing code, analysing data, and creating visualizations.
To start coding in Python, you need to set up Python correctly and choose an IDE that suits your needs. This will prepare you for your learning journey.
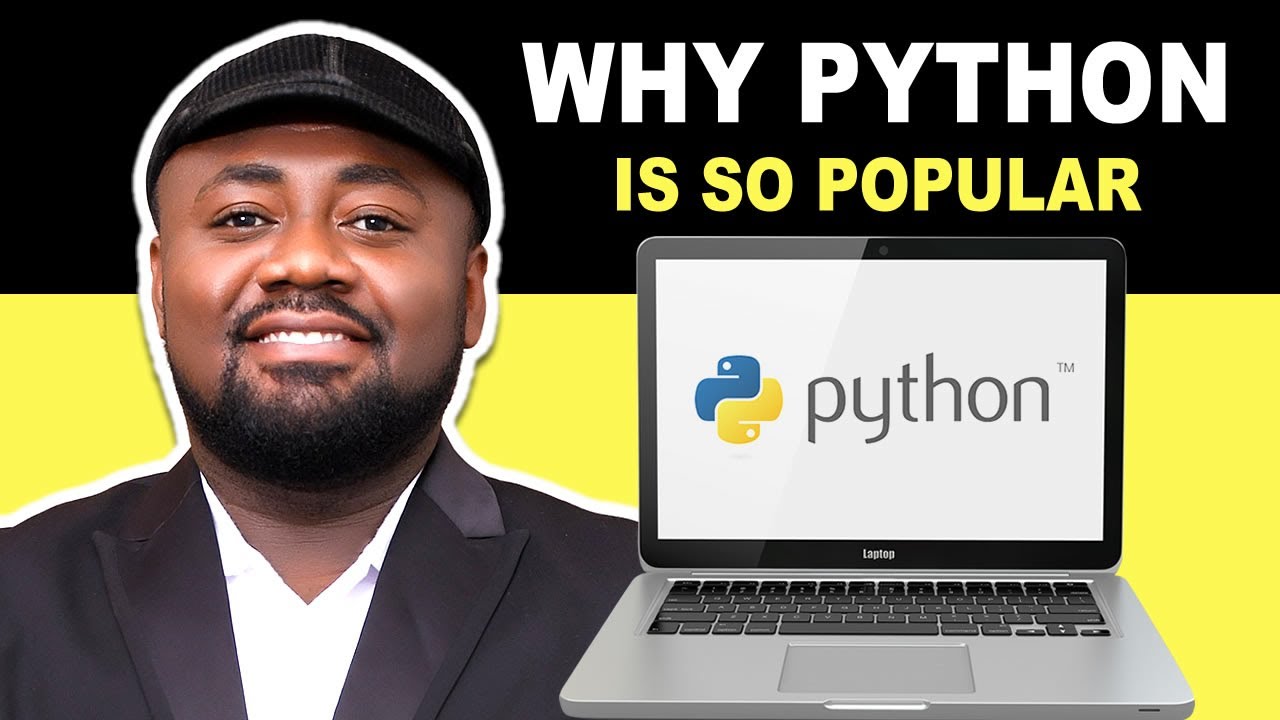
Basics of Python Syntax
Python is an interpreted, high-level, general-purpose programming language that is widely used for its simplicity and readability. If you are a beginner looking to learn the basics of Python, you’re in the right place!
Variables and data types in Python
In Python, variables are used to store values. Unlike some other programming languages, Python allows you to assign values to variables without explicitly declaring their data type. This means that variables can hold different types of data, such as integers, floating-point numbers, strings, booleans, and more.
Operators and expressions
Python provides a wide range of operators that can be used to perform various operations on variables and values. These operators include arithmetic operators (+, -, *, /), comparison operators (==, !=, <, >), logical operators (and, or, not), and more.
Expressions are combinations of variables, values, and operators that can be evaluated to produce a result. Python follows a specific order of precedence when evaluating expressions, but parentheses can be used to override this order if necessary.
Learning the basics of Python syntax will set you on the path to writing simple programs and solving problems. With practice and experience, you will gain a deeper understanding of Python’s capabilities and be able to tackle more complex projects.
Whether you’re interested in web development, data analysis, or machine learning, Python is a versatile language that can support your ambitions. So grab your computer and start exploring the world of Python programming today!
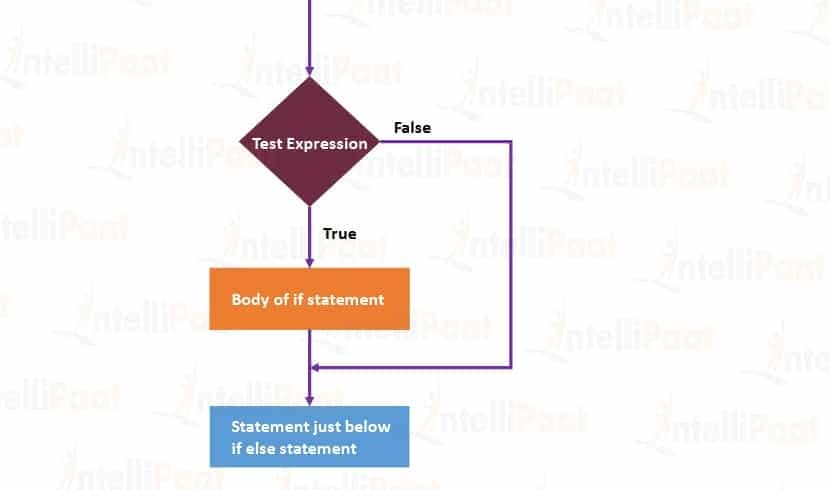
Control Flow Statements
Python is a popular programming language that is known for its simplicity and readability. For beginners, learning the basics of Python can be a great way to start their programming journey. One important aspect of Python is its control flow statements, which help in controlling the flow of execution within a program. Let’s take a closer look at two key control flow statements: conditional statements and loops.
Conditional statements (if, else, elif)
Conditional statements are used to perform different actions based on different conditions. The most commonly used conditional statement in Python is the if-else statement. You can use if-else to run a block of code when a condition is true and another block when the condition is false.
Another useful conditional statement is the elif statement, which allows you to check multiple conditions one after another. This is especially helpful when you have more than two possible outcomes.
Loops (for and while)
Loops are an essential part of any programming language as they allow you to execute a block of code repeatedly. In Python, there are two main types of loops: for loops and while loops.
A for loop iterates over a sequence (such as a list or string) or other iterable objects. It will execute the block of code for each item in the sequence.
On the other hand, a while loop will continue to execute a block of code as long as the specified condition remains true. This can be useful when you want to repeat an action until a certain condition is met.
Understanding control flow statements in Python is crucial for writing effective and efficient code. By mastering these concepts, beginners can gain more control over their programs and easily accomplish various tasks.
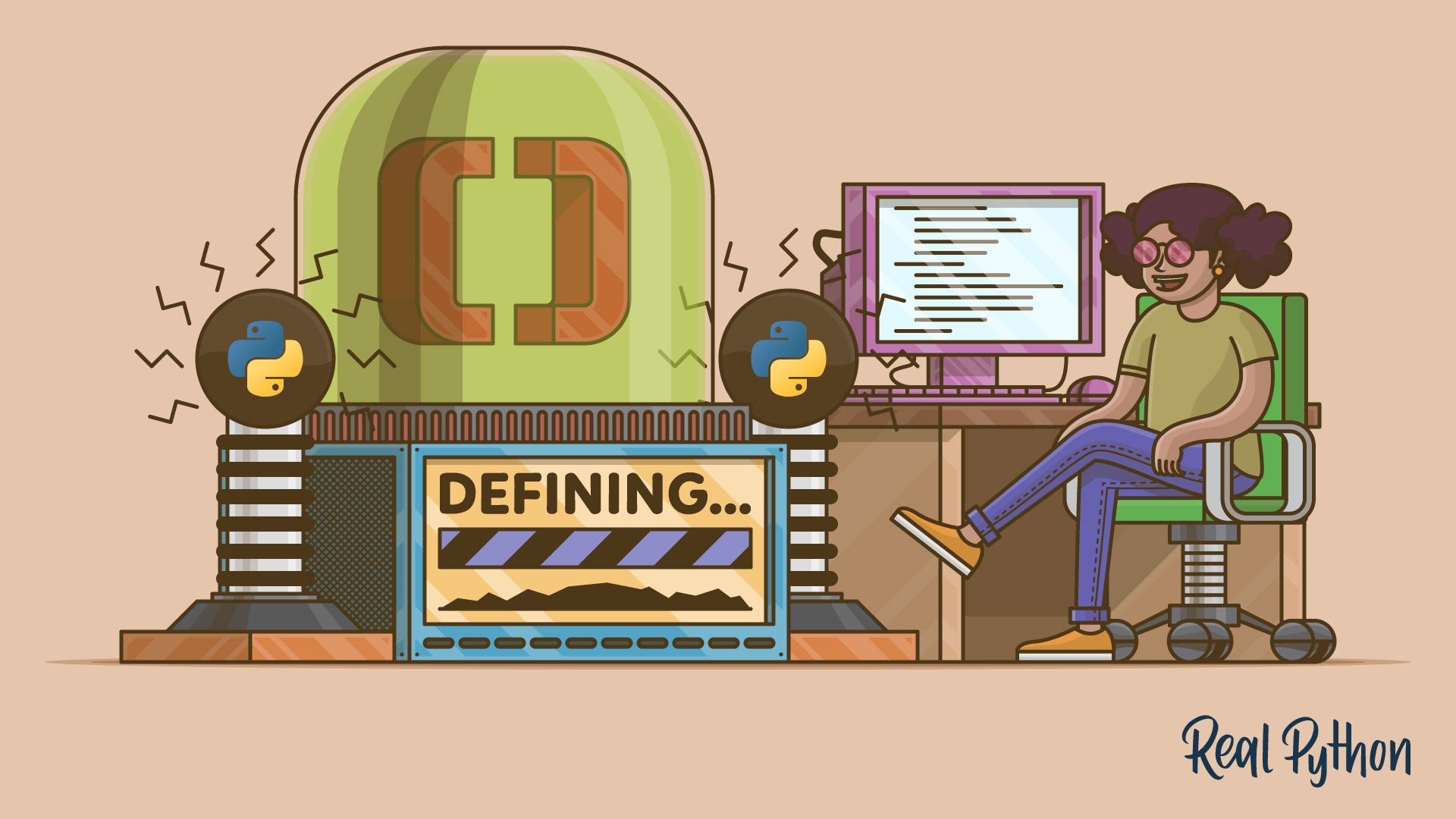
Functions in Python
Defining and calling functions
In Python, functions are blocks of code that perform specific tasks. They allow you to break down your program into smaller, more manageable pieces, making it easier to read, understand, and maintain.
To define a function in Python, you use the def keyword followed by the function name and parentheses. You can also include parameters inside the parentheses if the function requires any input values. Here’s an example:
def greet(): print("Hello, world!")
To call a function, write its name followed by parentheses. For example:
greet()
This will execute the code inside the function and print “Hello, world!” to the console.
Passing arguments and returning values
Functions can also accept arguments, which are values passed into the function to perform specific operations. You can specify these arguments when defining the function and use them within the function’s code.
Here’s an example of a function that takes two arguments and returns their sum:
def add_numbers(num1, num2): return num1 + num2
To use this function, you can pass in two numbers as arguments:
result = add_numbers(5, 3)print(result) # Output: 8
The function adds two numbers and returns the result. You can store it in a variable, print it, or use it for more calculations.
By understanding how to define functions, pass arguments, and return values in Python, you’ll have a solid foundation for building more complex programs.
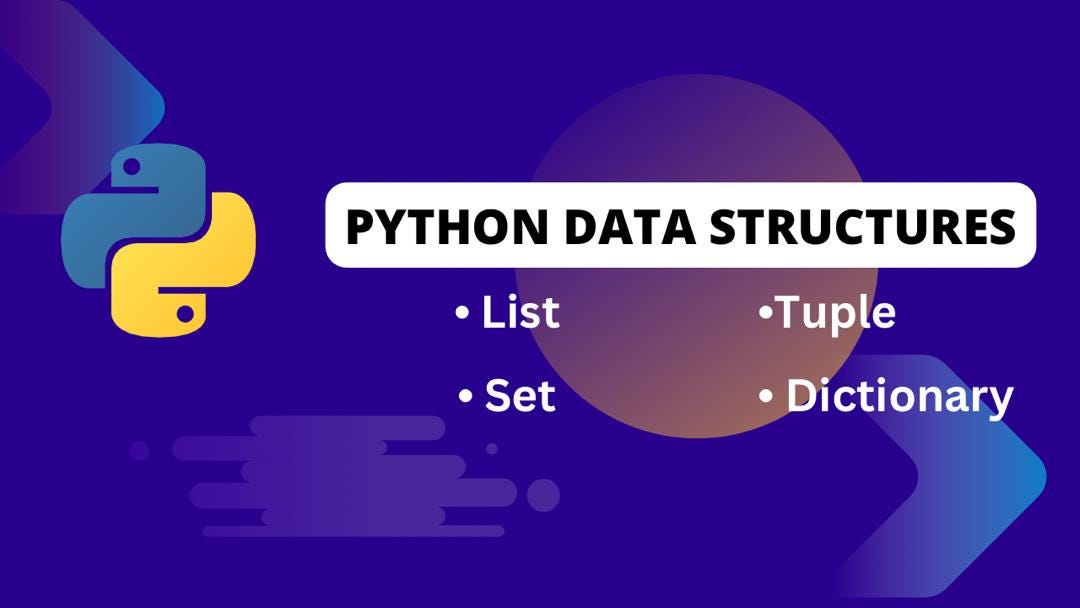
Data Structures in Python
When learning Python, it is essential to understand the various data structures it offers. Data structures are crucial for organizing and storing data in a way that allows for efficient access and manipulation.
Lists, tuples, and dictionaries
Lists: Lists are one of the most commonly used data structures in Python. They are mutable, meaning you can add, remove, and modify elements. Lists are denoted by square brackets [] and can store different types of elements such as integers, strings, or even other lists.
Tuples: Tuples are similar to lists but are immutable, meaning they cannot be changed once created. Tuples are denoted by parentheses () and are often used to store related pieces of information that should not be modified.
Dictionaries: Dictionaries are another useful data structure in Python. They consist of key-value pairs and allow for efficient retrieval of values based on their keys. Dictionaries are denoted by curly braces {} and offer flexibility in organizing and accessing data.
Accessing and manipulating data in data structures
Once you have created a data structure in Python, you can access and manipulate its data using various methods and operations.
Accessing data: To access specific elements within a data structure, you can use indexing or slicing. Indexing allows you to access a single element by its position, while slicing allows you to retrieve a range of elements.
Manipulating data: Python provides a wide range of built-in functions and methods for manipulating data in data structures. These include adding or removing elements, sorting, searching, and updating values.
Understanding Python’s data structures and how to access and manipulate their data is essential for creating complex programs and applications. So, dive into the world of Python and start exploring its powerful data structures!
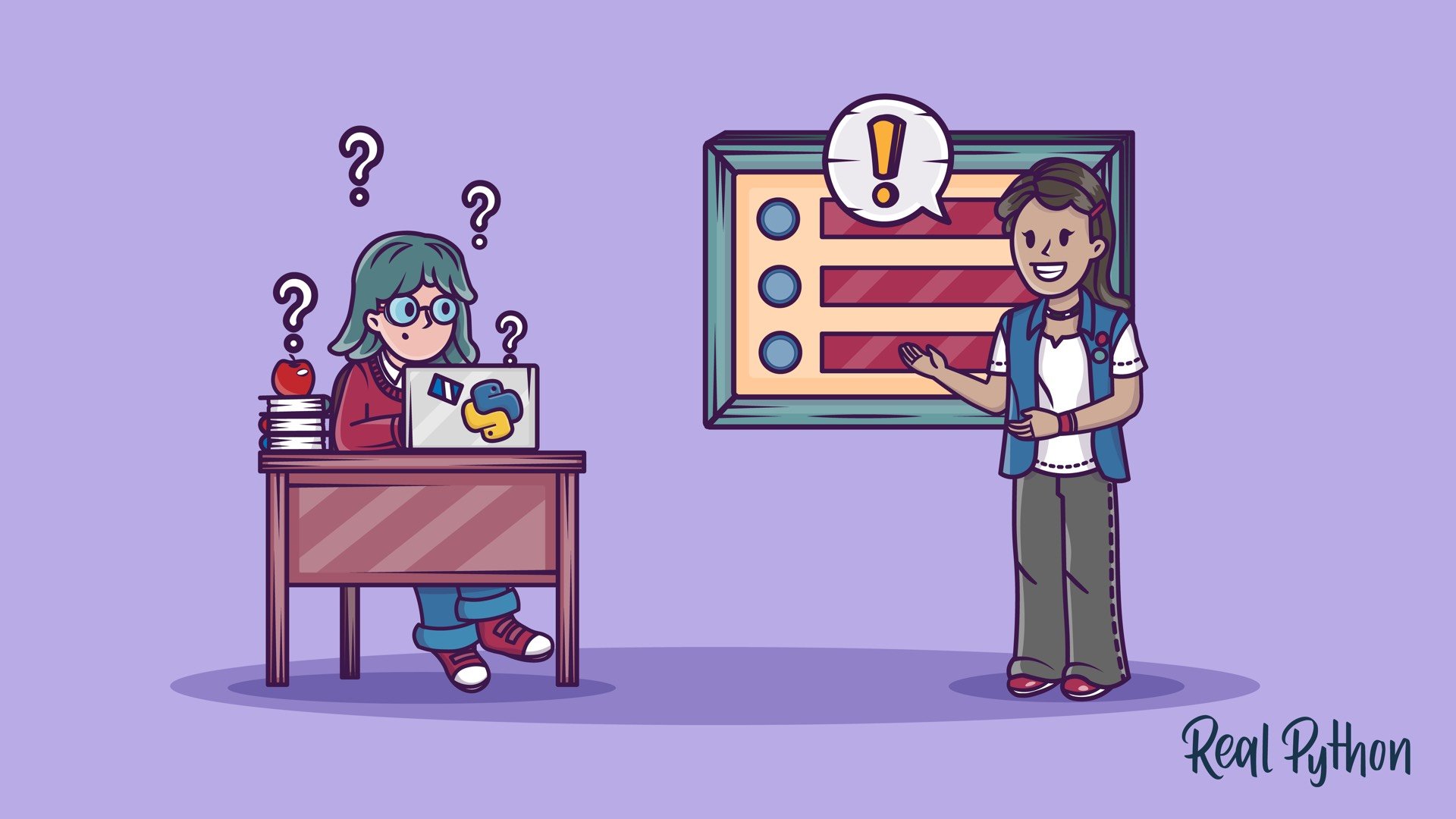
Input and Output Operations
Python, a widely-used programming language, offers intuitive and straightforward input and output operations mechanisms. These operations allow you to interact with files and users. This guide will cover two important aspects of input and output operations in Python: reading and writing files, and interacting with the user through input and output functionality.
Reading and writing files
Reading and writing files is a fundamental aspect of programming. Python provides simple and powerful tools for handling file operations. With just a few lines of code, you can read data from a file or write data to it. The open() function allows you to open files in various modes, such as read mode (‘r’), write mode (‘w’), append mode (‘a’), and more. You can also specify the file encoding if necessary.
Reading from a file involves using the read() or readlines() methods to extract the contents. The former reads the entire file as a single string, while the latter reads the file line by line into a list of strings.
On the other hand, writing to a file involves using the write() method to add content to the file. You can also use writelines() to write multiple lines at once.
Interacting with the user through input and output
Python allows you to interact with users by accepting their input and providing output based on that input. The input() function is used to receive user input as a string. You can then process this input and provide meaningful output based on your program’s logic.
Additionally, Python provides several built-in functions for common input/output tasks. For example, the print() function allows you to display information to the user. You can customize the output by formatting strings or using special characters like newline (‘\n’).
Mastering input and output operations in Python allows you to create programs that efficiently handle file data and provide a smooth user experience.
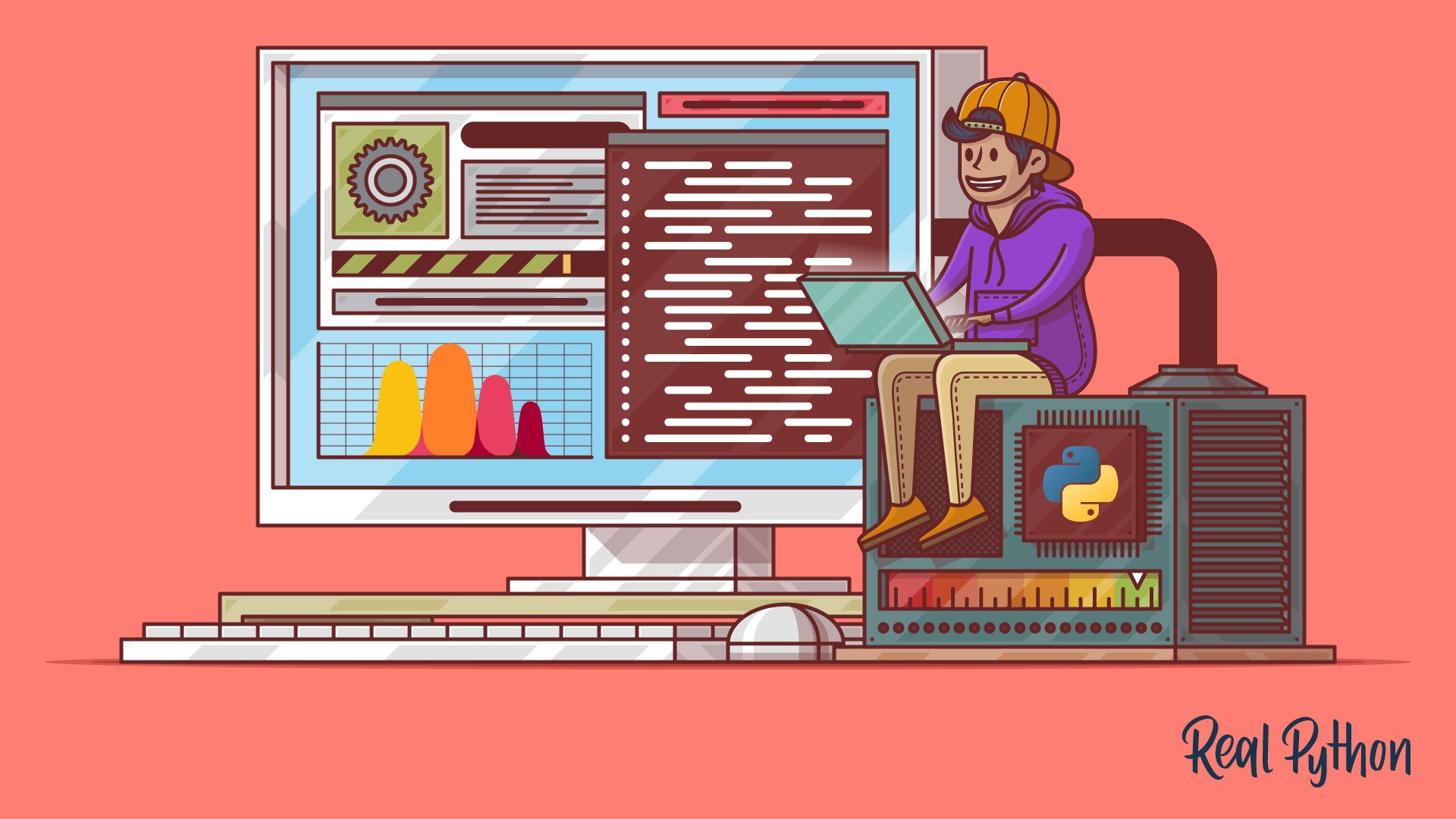
Error Handling and Exception Handling
When learning Python, understanding how to handle errors and exceptions is a crucial skill that every beginner must acquire. This step-by-step guide will walk you through the basics of error handling and exception handling in Python.
Understanding and handling errors and exceptions
What are errors and exceptions?
Errors occur when the program encounters a condition that it cannot deal with. Exceptions, on the other hand, are events that occur during the execution of a program that disrupts the normal flow of the program.
Why is error handling important?
Error handling allows you to gracefully handle unexpected errors or exceptional conditions that may occur during runtime. Without proper error handling, your program may crash or produce incorrect results, leading to a frustrating user experience.
Types of errors and exceptions
- Syntax errors: These occur when the code violates the rules of the Python language.
- Runtime errors: Also known as exceptions, these occur during the execution of a program due to various reasons such as division by zero, file not found, or improper input.
- Logical errors: These occur when there is a flaw in the design or logic of your code, resulting in incorrect output or undesired behavior.
How to handle errors and exceptions in Python
You can use the try-except block to handle errors and exceptions in Python. This block allows you to catch specific types of exceptions and execute alternative code or display error messages instead of crashing the program.
Using error handling and exception handling techniques in Python makes your programs robust, reliable, and user-friendly.
Conclusion and Next Steps
Congratulations on completing this step-by-step guide to learning the basics of Python for beginners! You now have an understanding of fundamental concepts such as variables, data types, conditionals, loops, and functions. With this foundation, you are well-equipped to explore more advanced topics and build upon your skills in Python programming.
Further resources for learning Python
While this guide has provided you with a solid starting point, there is always more to learn. Here are some additional resources that can help you continue your journey in mastering Python:
- Python.org: The official website of the Python programming language is a treasure trove of documentation, tutorials, and resources.
- Python Documentation: Dive deep into the official documentation to comprehensively understand Python’s features and capabilities.
- Online courses: Platforms like Udemy and Coursera offer a wide range of Python courses for beginners and advanced learners.
- Books: Numerous books are available on Python programming, catering to different skill levels. Some popular ones include “Python Crash Course” by Eric Matthes and “Learn Python the Hard Way” by Zed Shaw.
Summary of key concepts and next steps for practice
Recapping the key concepts covered in this guide:
- Variables store data that can be accessed and modified.
- Data types determine the kind of values variables can hold.
- Conditionals allow you to make decisions based on specific conditions.
- Loops enable you to repeat actions until a certain condition is met.
- Functions encapsulate reusable blocks of code.
To reinforce your understanding, it is recommended to practice coding exercises and work on small projects. The more you practice, the more confident you will become in writing Python code.
Keep exploring and experimenting with Python, and before you know it, you’ll be capable of developing sophisticated programs and applications. Happy coding!